
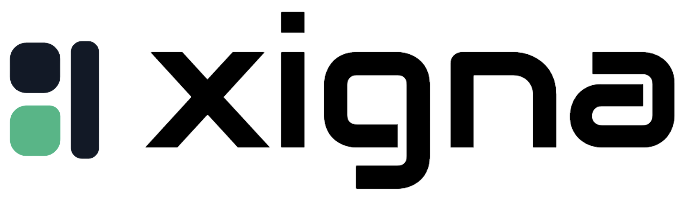
As the complexity of Django applications increases, so does the challenge of maintaining optimal performance, especially for data-intensive operations like fetching from APIs or rendering dynamic content. Slow initial load times can deter users and hamper user experience. Caching is a potent solution to mitigate these challenges by storing frequently accessed data for quick retrieval. This article delves into caching techniques in Django, including pre-warming the cache and its implications on performance.
Understanding the Challenge:
Picture a scenario where a Django app retrieves data from an external API, such as blog posts. Upon the initial request, the application must fetch data from the API, resulting in slower response times. This latency persists for subsequent requests if not managed effectively.
The Role of Caching:
Caching acts as a performance booster by storing data in memory or disk, reducing the need for repetitive requests to external sources. It enhances response times, diminishes server load, and ultimately enhances user experience, especially crucial for applications with high traffic or heavy reliance on external APIs.
Pre-Warming the Cache:
Pre-warming the cache involves populating it with data before it's requested, ensuring it's readily available when needed. Django facilitates this process during application startup using a custom AppConfig.
Code Snippet - Pre-Warming the Cache:
python
# myapp/apps.py from django.apps import AppConfig from .cache_utils import prewarm_cache class MyAppConfig(AppConfig): name = 'myapp' def ready(self): prewarm_cache()
Implementing Caching in Views:
Django offers decorators like cache_page
to cache view responses for a specified duration. This technique enables subsequent requests for the same data to be served directly from the cache, significantly boosting response times.
Code Snippet - Caching in Django Views:
python
# myapp/views.py from django.views.decorators.cache import cache_page @cache_page(60 * 60 * 24 * 7) # Cache for 7 days def my_view(request): # Your view logic here
Balancing Act:
While caching yields performance benefits, it comes with caveats. Stale data is a primary concern, as outdated cache entries may present obsolete information to users until refreshed. Moreover, caching can strain memory resources, particularly with large datasets or prolonged cache durations.
Conclusion:
In summary, caching serves as a potent weapon in the Django developer's arsenal, mitigating slow response times and optimizing user experience. By pre-warming the cache and strategically caching views, developers can ensure swift initial load times and overall application responsiveness. Nevertheless, it's imperative to navigate the trade-offs wisely and employ cache invalidation strategies to combat stale data issues. With a well-structured caching strategy, Django applications can strike the perfect balance between performance enhancement and data integrity.